How to add an x-offset to a text pattern with every x-step?
I am creating a text pattern within a rectangle. Is there a way to increase the xStep
parameter of the pattern?
I am creating a text pattern within a rectangle with the following snippet:
var canvas = m_writer.DirectContent;
float fillTextSize = 6.0f;
string filltext = "this is the fill text!";
float filltextWidth = m_dejavuMonoBold.GetWidthPoint(filltext, fillTextSize);
PdfPatternPainter pattern = canvas.CreatePattern(px, py, filltextWidth, fillTextSize);
pattern.BeginText();
pattern.SetTextMatrix(0, 0);
pattern.SetTextRenderingMode(PdfContentByte.TEXT_RENDER_MODE_FILL);
pattern.SetRGBColorStroke(190, 190, 190);
pattern.SetRGBColorFill(190, 190, 190);
pattern.SetFontAndSize(m_dejavuMonoBold, 6.0f);
pattern.ShowText(filltext);
pattern.EndText();
canvas.Rectangle(px, py, pw, ph);
canvas.SetPatternFill(pattern);
canvas.Fill();
The fill text is repeated regularly. I want to add with every printed line of the pattern to add a horizontal offset. Is there a way to increase the xStep
parameter of the pattern?
I am looking for a pattern like this:
|this is the fill text! this is the fill text! this|
|his is the fill text! this is the fill text! this |
|is is the fill text! this is the fill text! this i|
|s is the fill text! this is the fill text! this is|
| is the fill text! this is the fill text! this is |
Posted on StackOverflow on Jul 7, 2015 by Code Clown
If I understand your question correctly, you need something like this: text_pattern.pdf
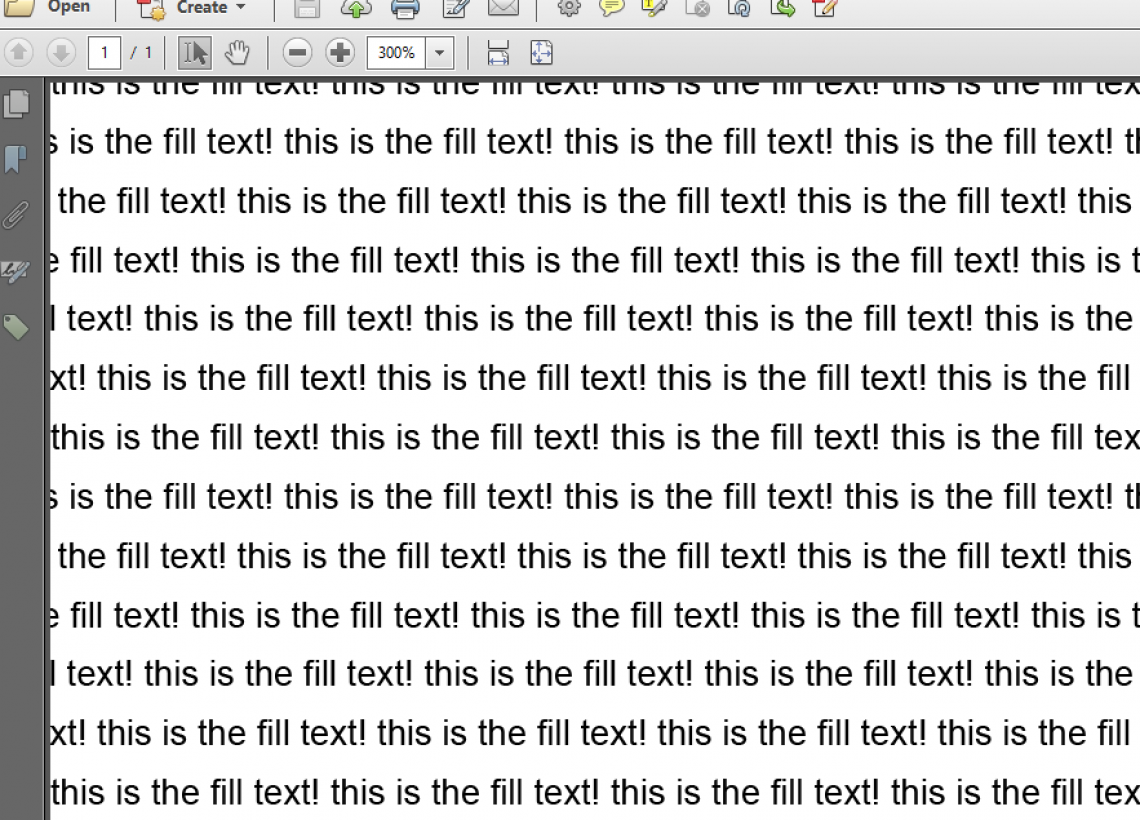
Text pattern example
We are using tiled patterns and the problem you experience is that all tiles are regular. If you want an irregular pattern, you need to use a workaround. Such a workaround is shown in the TextPattern example.
In iText 7 there is a Tiling
class with a constructor for creating a tile. Declaring pattern you can set the width, height, X and Y offsets (like PdfPatternPainter
in iText 5). Then you should create a PdfPatternCanvas
using your tile:
PdfPattern.Tiling tilingPattern = new PdfPattern.Tiling(filltextWidth, 60, filltextWidth, 60);
PdfPatternCanvas patternCanvas = new PdfPatternCanvas(tilingPattern, pdf);
patternCanvas.beginText().setFontAndSize(font, 6.f);
// Start with an X offset of 0
float x = 0;
// Add 6 rows of text
// The font is 6, so use a leading of 10:
for (float y = 0; y < 60; y += 10) {
// Add the same text twice to each row
// 1:
patternCanvas.setTextMatrix(x - filltextWidth, y);
patternCanvas.showText(filltext);
// 2:
patternCanvas.setTextMatrix(x, y);
patternCanvas.showText(filltext);
// Change the X offset to 1/6th of the text width
x += (filltextWidth / 6);
}
patternCanvas.endText();
As far as I know, adding the text more than once to the pattern, is the only way to achieve the type of pattern you require.
Click How to add an x-offset to a text pattern with every x-step? if you want to see how to answer this question in iText 5.