How to add an x-offset to a text pattern with every x-step?
I am creating a text pattern within a rectangle. Is there a way to increase the xStep
parameter of the pattern?
I am creating a text pattern within a rectangle with the following snippet:
var canvas = m_writer.DirectContent;
float fillTextSize = 6.0f;
string filltext = "this is the fill text!";
float filltextWidth = m_dejavuMonoBold.GetWidthPoint(filltext, fillTextSize);
PdfPatternPainter pattern = canvas.CreatePattern(px, py, filltextWidth, fillTextSize);
pattern.BeginText();
pattern.SetTextMatrix(0, 0);
pattern.SetTextRenderingMode(PdfContentByte.TEXT_RENDER_MODE_FILL);
pattern.SetRGBColorStroke(190, 190, 190);
pattern.SetRGBColorFill(190, 190, 190);
pattern.SetFontAndSize(m_dejavuMonoBold, 6.0f);
pattern.ShowText(filltext);
pattern.EndText();
canvas.Rectangle(px, py, pw, ph);
canvas.SetPatternFill(pattern);
canvas.Fill();
The fill text is repeated regularly. I want to add with every printed line of the pattern to add a horizontal offset. Is there a way to increase the xStep
parameter of the pattern?
I am looking for a pattern like this:
|this is the fill text! this is the fill text! this|
|his is the fill text! this is the fill text! this |
|is is the fill text! this is the fill text! this i|
|s is the fill text! this is the fill text! this is|
| is the fill text! this is the fill text! this is |
Posted on StackOverflow on Jul 7, 2015 by Code Clown
If I understand your question correctly, you need something like this: text_pattern.pdf
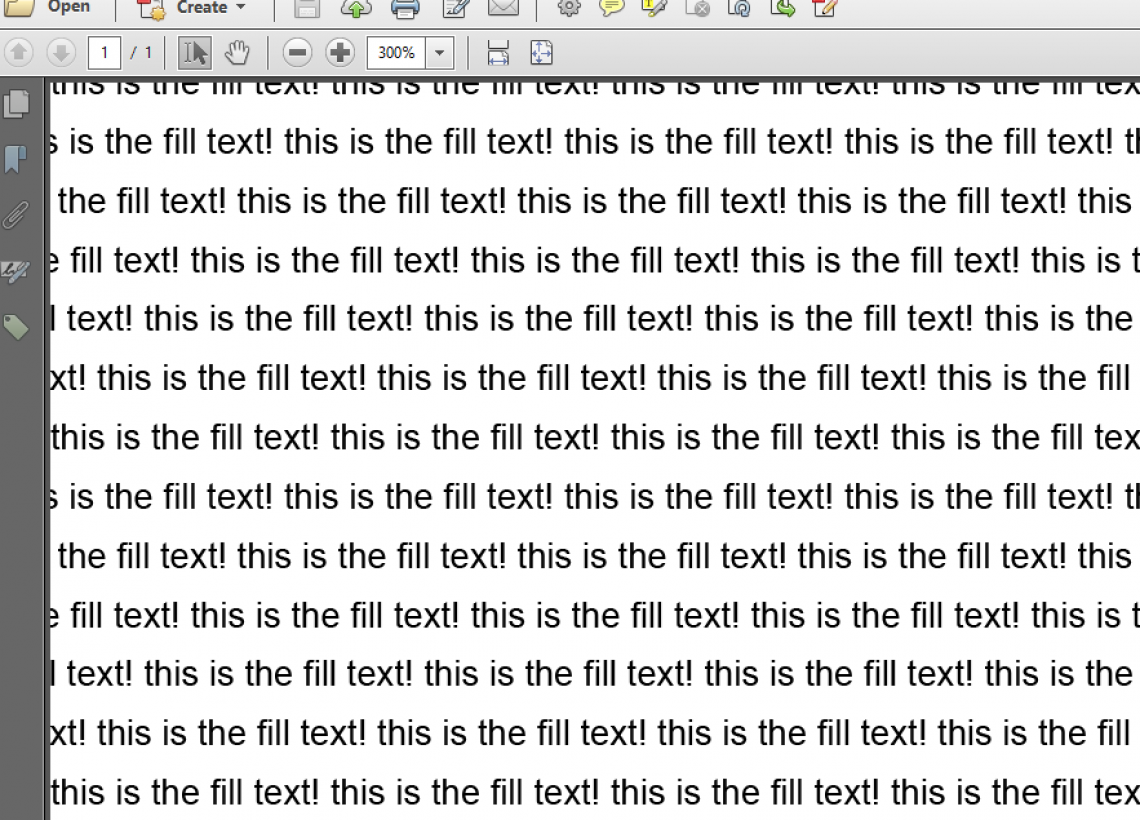
Text pattern example
We are using tiled patterns and the problem you experience is that all tiles are regular. If you want an irregular pattern, you need to use a workaround. Such a workaround is shown in the TextPattern example.
In this example, I create the pattern like this:
// This corresponds with what you have
PdfContentByte canvas = writer.getDirectContent();
BaseFont bf = BaseFont.createFont();
String filltext = "this is the fill text! ";
float filltextWidth = bf.getWidthPoint(filltext, 6);
// I create a bigger "tile"
PdfPatternPainter pattern = canvas.createPattern(filltextWidth, 60, filltextWidth, 60);
pattern.beginText();
pattern.setFontAndSize(bf, 6.0f);
// I start with an X offset of 0
float x = 0;
// I add 6 rows of text
// The font is 6, so I used a leading of 10:
for (float y = 0; y < 60; y += 10) {
// I add the same text twice to each row
// 1.
pattern.setTextMatrix(x - filltextWidth, y);
pattern.showText(filltext);
// 2.
pattern.setTextMatrix(x, y);
pattern.showText(filltext);
// I change the X offset to 1/6th of the text width
x += (filltextWidth / 6);
}
pattern.endText();
As far as I know, adding the text more than once to the pattern, is the only way to achieve the type of pattern you require.