How to add / delete / retrieve information from a PDF using a custom property?
I want to store 2 strings (string1
and string2
) as a custom entry (key and value) that is stored inside the PDF in the custom property area. You can find this custom property area under File > Properties > Custom > Custom properties in Adobe Reader, where you can find "Name" and "Value" in pairs. I want to store string1
in the "Value" and store string2
in the "Name". Later, I want to retrieve/delete the strings in the custom property area.
Posted on StackOverflow on Nov 4, 2014 by brian
In order to better understand the problem, I've used Acrobat to add a custom metadata entry named "Test" with value "test" and when you look inside that file, you can see that this key/value pair turns up on two places (marked with a red dot).
It is present in the Info dictionary, which is the traditional place to store metadata.
It is present in the XMP metadata stream as a tag named Test with prefix pdfx (for custom tags).
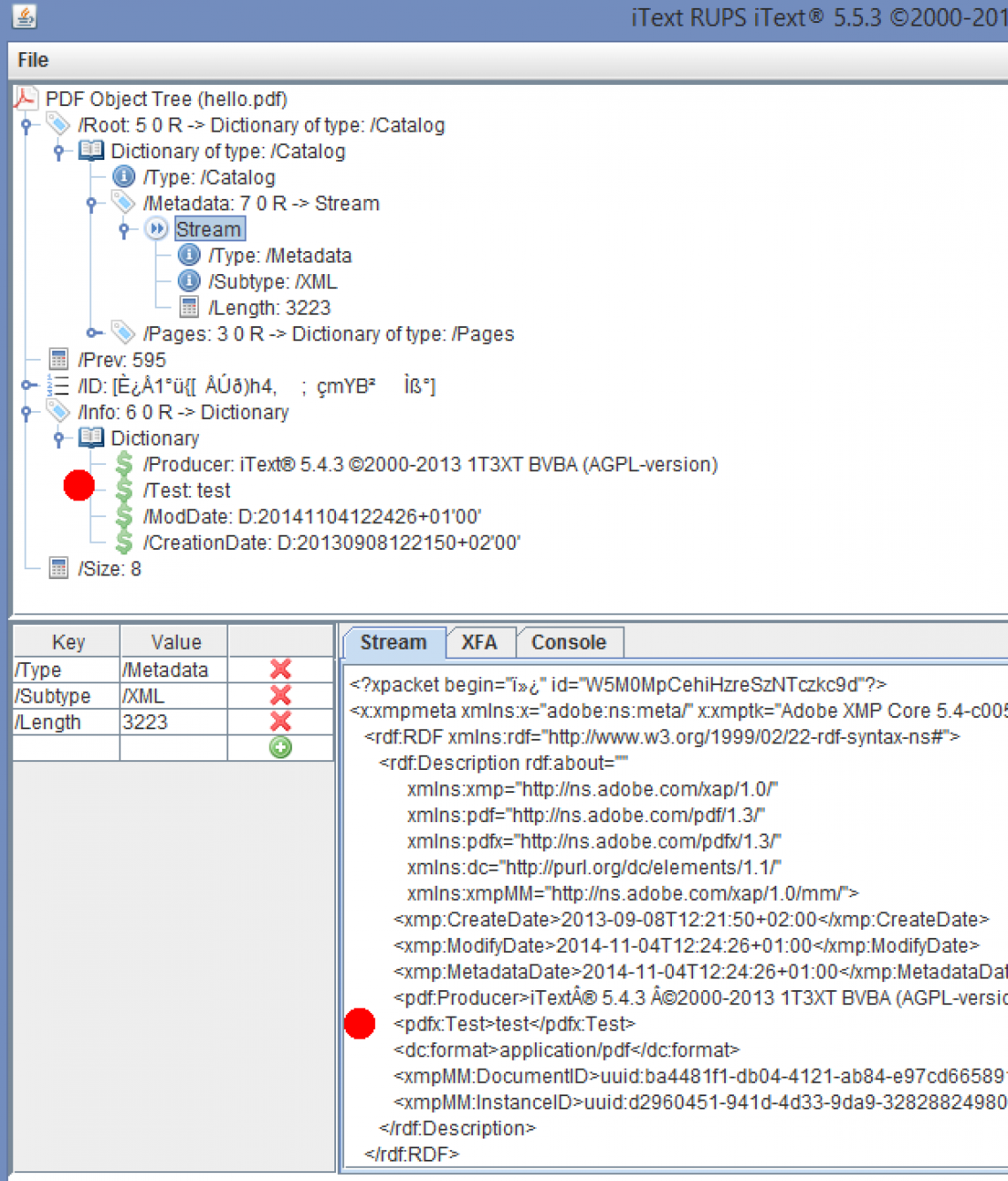
Metadata inside a PDF file
Adding an extra value to the Info dictionary is easy when using iText. Updating the XMP metadata is also possible, but you'll have to create the XMP stream yourself and maybe it's overkill in your case. Maybe your PDF only has an Info dictionary and no XMP.
Moreover: you say that the purpose of having that key is to retrieve its value and to delete the custom entry afterwards. In that case, it's sufficient to add the extra entry in the Info dictionary.
Depending on whether you want to add a custom entry to the Info dictionary to a PDF created from scratch or to an existing PDF you need one of the following examples.
In CustomMetaEntry, we add a standard metadata entry for the title and a custom entry, Test.
public void createPdf(String dest) throws IOException, DocumentException {
Document document = new Document();
PdfWriter.getInstance(document, new FileOutputStream(dest));
document.addTitle("Some example");
document.add(new Header("Test", "test"));
document.open();
Paragraph p = new Paragraph("Hello World");
document.add(p);
document.close();
}
As you can see, iText had addX()
methods to add Title, Author,... metadata. However, if you want to add a custom entry, you need to use the add()
method to add a Header
instance. You need to add the metadata before opening the document.
If you want to add entries to the info dictionary of an existing PDF, take a look at the MetadataPdf example:
public void manipulatePdf(String src, String dest) throws IOException, DocumentException {
PdfReader reader = new PdfReader(src);
PdfStamper stamper = new PdfStamper(reader, new FileOutputStream(dest));
Map info = reader.getInfo();
info.put("Title", "Hello World stamped");
info.put("Subject", "Hello World with changed metadata");
info.put("Keywords", "iText in Action, PdfStamper");
info.put("Creator", "Silly standalone example");
info.put("Author", "Also Bruno Lowagie");
stamper.setMoreInfo(info);
stamper.close();
reader.close();
}
In this example, we get the info dictionary from a PdfReader
instance using the getInfo()
method. This also answers how to retrieve the custom data from a PDF. If the Map
contains an entry with key Test
, you can get its value like this:
String test = info.get("Test");
You can now add extra pairs of String
s to this Map
. In the example, we add standard keys for metadata, but you can also use custom keys.
Removing an entry from an existing PDF file is done in the same way as adding an entry. It's sufficient to add a null
value. For instance:
info.put("Test", null);
This will remove a custom entry named Test
in case such a value was present in your Info dictionary.